在 Aerial Data Lake 数据上使用 pyAerial 进行信道估计#
此示例展示了如何使用 pyAerial 绑定来运行 cuPHY GPU 加速的 5G NR PUSCH 信道估计。5G NR PUSCH 数据从示例空中捕获的 PUSCH 数据集中读取,该数据集使用 Aerial Data Lake 收集和存储,并且信道是使用 pyAerial 和基于相应 PUSCH 参数的 cuPHY 估计的。
注意: 此示例需要 clickhouse 服务器正在运行,并且示例数据已存储在数据库中。请参阅 Aerial Data Lake 文档,了解如何执行此操作。
[1]:
# Check platform.
import platform
if platform.machine() not in ['x86_64', 'aarch64']:
raise SystemExit("Unsupported platform!")
导入#
[2]:
import math
import os
os.environ["CUDA_VISIBLE_DEVICES"] = "0"
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# Connecting to clickhouse on remote server
import clickhouse_connect
# Import the channel estimator and some utilities for converting
# the DMRS fields in the right format from the SCF FAPI format that the dataset follows.
from aerial.phy5g.algorithms import ChannelEstimator
from aerial.util.fapi import dmrs_fapi_to_bit_array
数据#
我们使用从真实空中 PUSCH 传输中捕获的示例数据集。数据库中的“fapi”表包含每个 PUSCH 传输的元数据,而“fh”表包含该时隙的所有样本。
[3]:
# Create the pyAerial (cuPHY) channel estimator.
num_ues = 1
num_rx_ant = 4
channel_estimator = ChannelEstimator(num_rx_ant=num_rx_ant)
# Connect to the local database
client = clickhouse_connect.get_client(host='localhost')
# Pick some pusch records from the database
pusch_records = client.query_df('select * from fapi order by TsTaiNs limit 10')
运行信道估计#
从 PUSCH 记录中,我们提取 PUSCH DMRS 参数,并使用 TAI 时间条目选择该时隙的 IQ 样本。然后使用提取的参数运行信道估计,并将估计信道的绝对值绘制在同一图中。
[4]:
for index,pusch_record in pusch_records.iterrows():
query = f"""select TsTaiNs,fhData from fh where
TsTaiNs == {pusch_record.TsTaiNs.timestamp()}
"""
fh = client.query_df(query)
# Make sure that the fronthaul database is complete for the SFN.Slot we've chosen
if fh.index.size < 1:
pusch_records = pusch_records.drop(index)
continue;
fh_samp = np.array(fh['fhData'][0], dtype=np.float32)
rx_slot = np.swapaxes(fh_samp.view(np.complex64).reshape(4, 14, 273*12),2,0)
# Extract all the needed parameters from the PUSCH record.
slot = int(pusch_record.Slot)
rntis = [pusch_record.rnti]
layers = [pusch_record.nrOfLayers]
start_prb = pusch_record.rbStart
num_prbs = pusch_record.rbSize
start_sym = pusch_record.StartSymbolIndex
num_symbols = pusch_record.NrOfSymbols
scids = [int(pusch_record.SCID)]
data_scids = [pusch_record.dataScramblingId]
dmrs_scrm_id = pusch_record.ulDmrsScramblingId
num_dmrs_cdm_grps_no_data = pusch_record.numDmrsCdmGrpsNoData
dmrs_syms = dmrs_fapi_to_bit_array(int(pusch_record.ulDmrsSymbPos))
dmrs_ports = [pusch_record.dmrsPorts]
dmrs_max_len = 1
dmrs_add_ln_pos = 2
num_subcarriers = num_prbs * 12
mcs_tables = [pusch_record.mcsTable]
mcs_indices = [pusch_record.mcsIndex]
coderates = [pusch_record.targetCodeRate/10.]
tb_sizes = [pusch_record.TBSize]
mod_orders = [pusch_record.qamModOrder]
tb_input = np.array(pusch_record.pduData)
# Run PyAerial (cuPHY) channel estimation.
ch_est = channel_estimator.estimate(
rx_slot=rx_slot,
num_ues=num_ues,
layers=layers,
scids=scids,
slot=slot,
dmrs_ports=dmrs_ports,
dmrs_syms=dmrs_syms,
dmrs_scrm_id=dmrs_scrm_id,
dmrs_max_len=dmrs_max_len,
dmrs_add_ln_pos=dmrs_add_ln_pos,
num_dmrs_cdm_grps_no_data=num_dmrs_cdm_grps_no_data,
start_prb=start_prb,
num_prbs=num_prbs,
prg_size=1,
num_ul_streams=1,
start_sym=start_sym,
num_symbols=num_symbols
)
fig, axs = plt.subplots(1)
fig.suptitle("Channel estimates for SFN.Slot: "+str(pusch_record.SFN)+"."+str(pusch_record.Slot))
axs.set_title(pusch_record.TsTaiNs)
for ant in range(4):
axs.plot(np.abs(ch_est[0][ant, 0, :, 0]))
axs.grid(True)
plt.show()
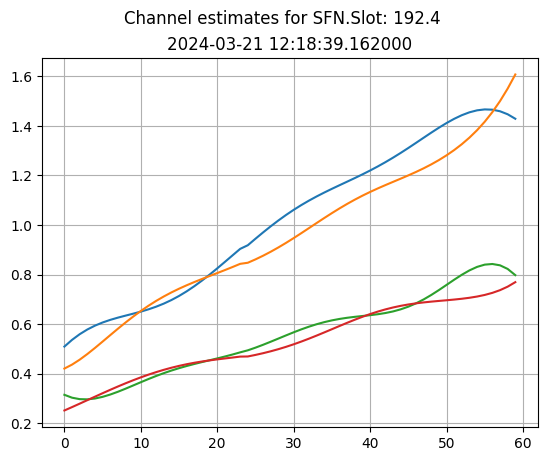
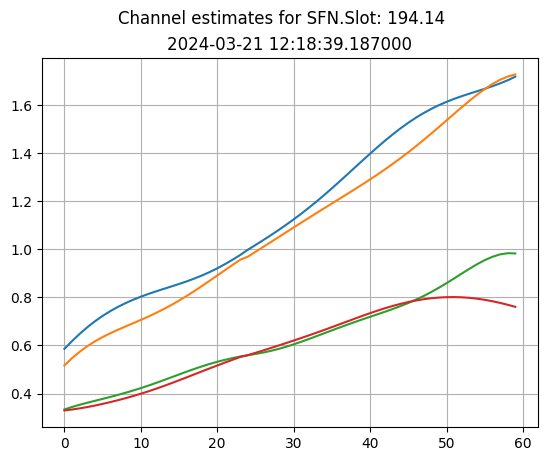
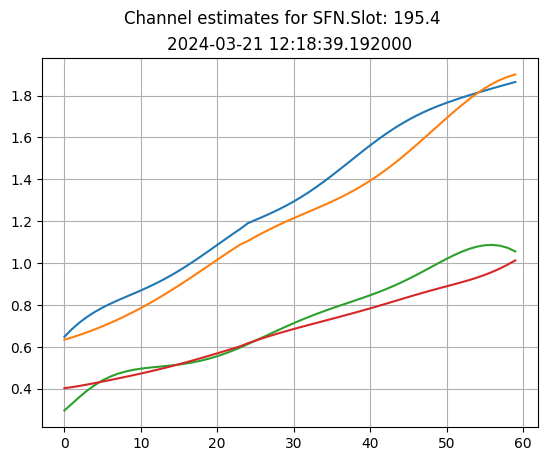
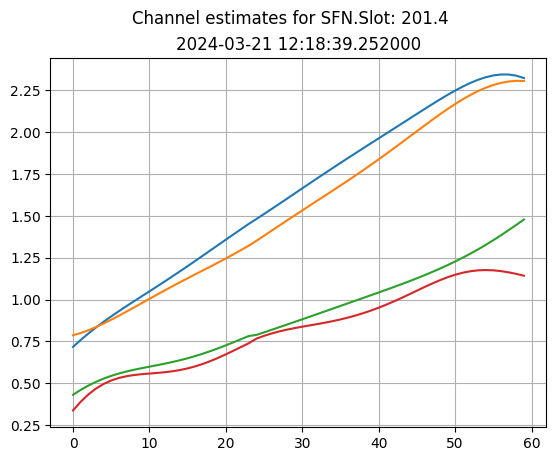
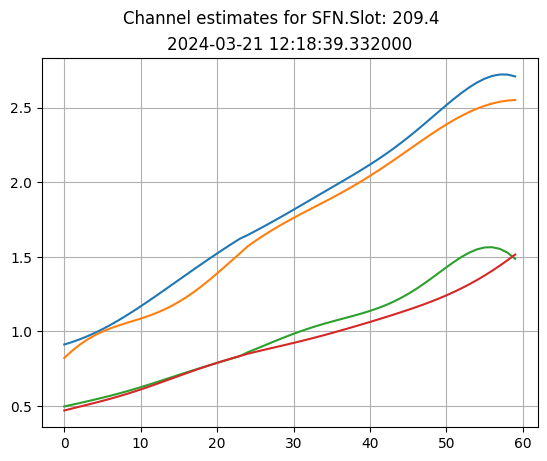