概述
此示例展示了 VPI 和 PyTorch 之间在 Python 中的互操作性如何工作。它展示了一组简单的图像处理操作,应用于 PyTorch 和 VPI 中的示例输入图像,而无需在互操作期间进行任何内存复制。
此示例使用 PyTorch 作为示例,但是,这种互操作性可以与任何支持 cuda 数组接口的库一起使用。
诸如以下的库
该示例从您提供的图像开始,将其转换为灰度图像,然后对其执行以下操作序列
- 使用 PyTorch 降低图像强度
- 执行 PyTorch -> VPI 互操作性,无需内存复制
vpi_image = vpi.asimage(torch_image)
- 使用 VPI 应用盒状滤波器
- 执行 VPI -> PyTorch 互操作性,无需内存复制
with vpi_image.rlock_cuda() as cuda_buffer
torch_image = torch.as_tensor(cuda_buffer)
或者,使用额外的深度内存复制torch_image = torch.as_tensor(vpi_image.cuda())
- 使用 PyTorch 增加图像强度
然后将结果保存到磁盘。
说明
用法是
其中
- 输入图像:输入图像文件名;它接受 png、jpeg 以及可能的其他格式。
这是一个示例
- Python
python3 main.py ../assets/kodim08.png
该示例将生成 vpi_pytorch.png
作为输出文件。
结果
输入图像 | 输出图像 |
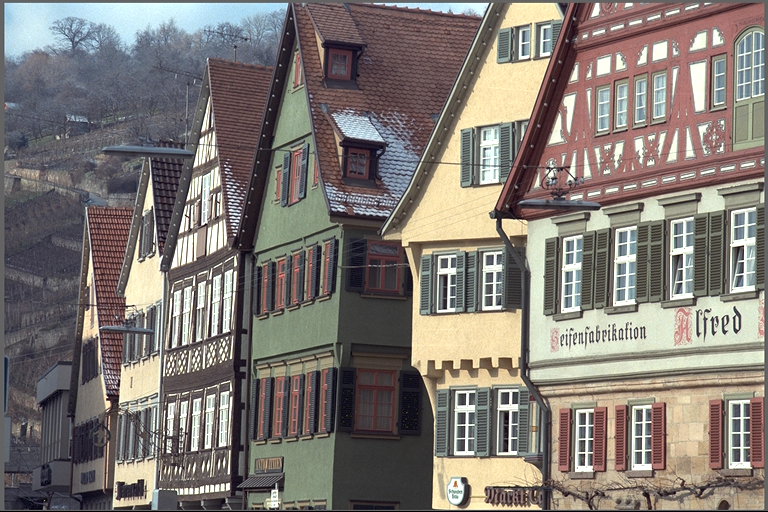 | |
源代码
为了方便起见,以下代码也安装在示例目录中。
31 from PIL
import Image, ImageOps
32 from argparse
import ArgumentParser
35 parser = ArgumentParser()
36 parser.add_argument(
'input', help=
'Image to be used as input')
38 args = parser.parse_args()
41 assert torch.cuda.is_available()
42 cuda_device = torch.device(
'cuda')
46 pil_image = ImageOps.grayscale(Image.open(args.input))
48 sys.exit(
"Input file not found")
50 sys.exit(
"Error with input file")
53 np_image = np.asarray(pil_image)
56 torch_image = torch.asarray(np_image).cuda()
59 torch_image = torch_image/255 * 0.5
62 vpi_image = vpi.asimage(torch_image)
67 vpi_image = vpi_image.box_filter(3)
70 with vpi_image.rlock_cuda()
as cuda_buffer
72 torch_tensor = torch.as_tensor(cuda_buffer, device=cuda_device)
73 torch_image = torch_tensor*255 + 128
76 np_image = np.asarray(torch_image.cpu())
79 pil_image = Image.fromarray(np_image)
82 pil_image.convert(
'L').save(
'vpi_pytorch.png')